2019. 11. 14. 22:51ㆍ🔴 Spring
DispatcherServelt API = 프론트 Controller패턴
프론트 Controller 프로세스:
"중앙 집중형 Controller", 프리젠테이션 계층의 앞단에 놓음, 예외 → 일관된 방식으로 처리
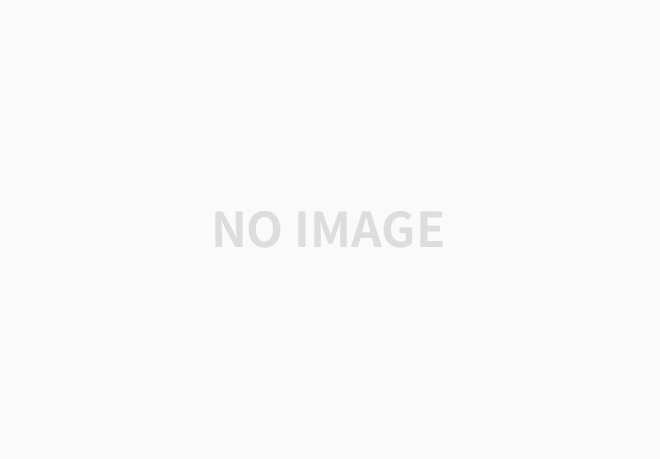
- 클라이언트가 보낸 요청 → 공통적인 작업 먼저 수행
- 세부 Controller로 작업 위임
- View 선택 → 최종결과 생성
-
모든 요청에 대하여 공통적으로 처리되어야 하는 로직 → 중앙 집중적으로 클라이언트 요청 관리함
-
Spring MVC 구성요소 이용 : controller (핵심처리, view이름 반환) & view
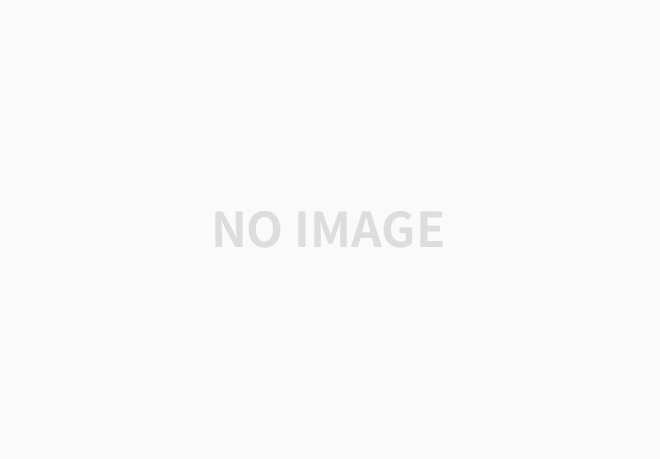
** 스프링 컨테이너 (WebApplicationContext)를 자동생성하기 때문에
전에 DI에서 main method → application context따위를 new로 생성하지 않아도 된다
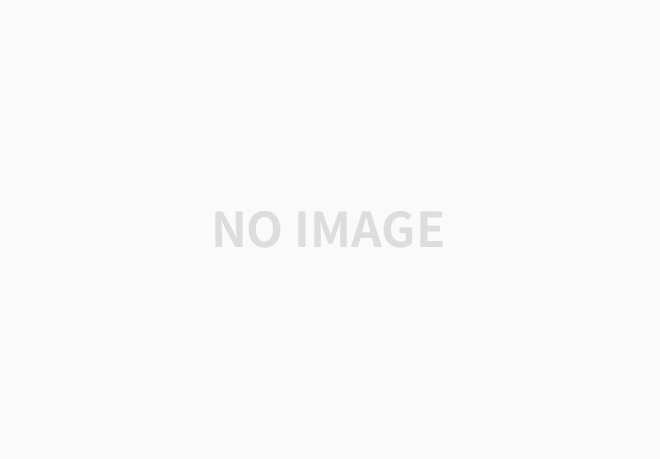
스프링 컨테이너(WebApplicationContext)생성 → 필요한 Bean 객체 구함
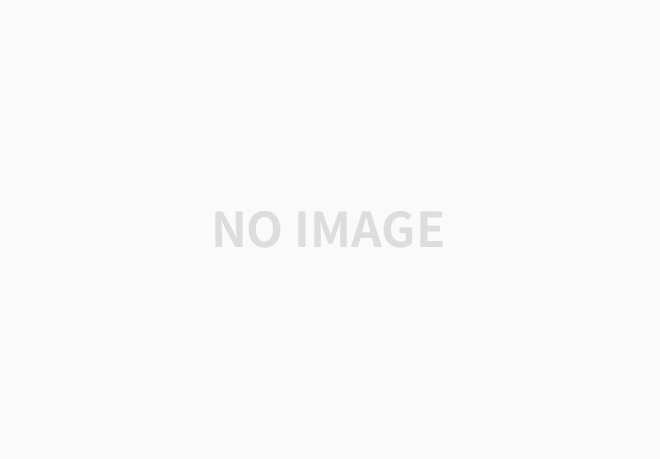
HandlerMapping
<구성요소>
SimpleUrlHandlerMapping | URL과 컨트롤러 이름을 직접 매핑 한다. |
BeanNameUrlHandlerMapping | URL과 일치하는 이름을 갖는 빈을 컨트롤러로 사용한다. |
ControllerClassNameHandlerMapping | URL과 매칭되는 클래스 이름을 갖는 빈을 컨트롤러로 사용한다. |
DefaultAnnotationHandlerMapping | @RequestMapping 어노테이션을 이용하여 컨트롤러와 매핑한다. |
--> @RequestMapping("경로")
경로 → 앞에 "/"써주기!! 안써줘도 실행은 되지만 나중에 세부 RequestMapping 추가적으로 밑에서 설정해줄때 헷갈리지 않도록 하기 위해서 써준다.
ex. @RequestMapping("/member/memberLogin/") + 클래스
@RequestMapping("list") + 메소드
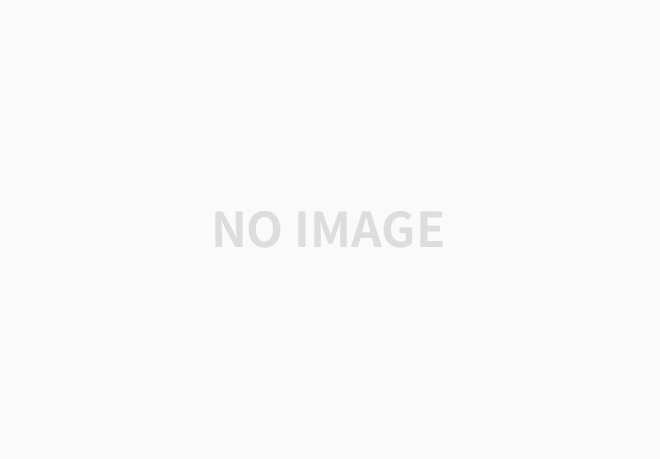
개발자가 컨트롤해야하는 부분 : Controller, view name, ⇒ 에 따라서 view 달라짐
DispatcherServlet : Spring의 내부적인 구조
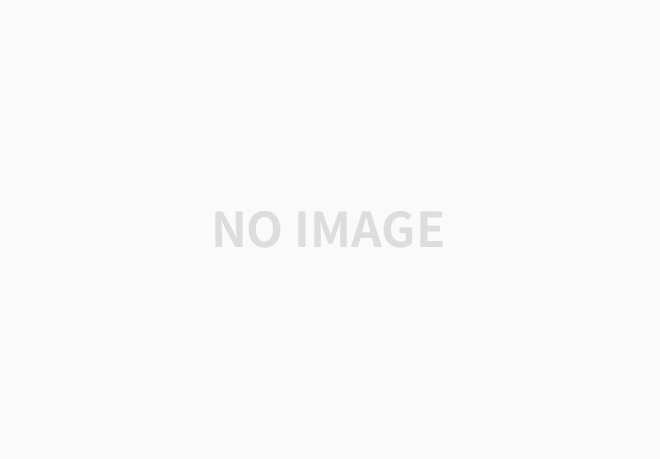
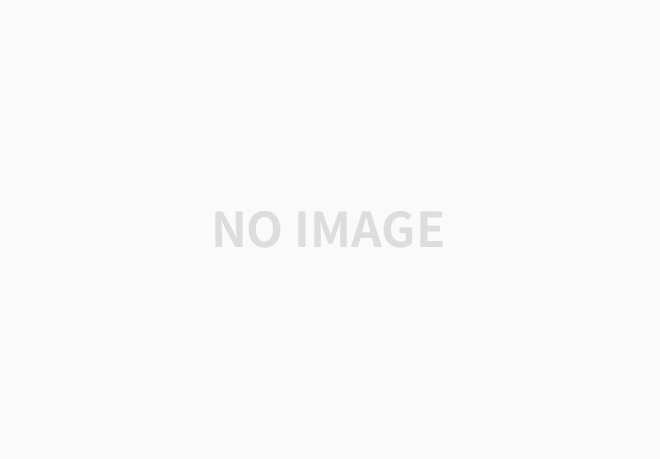
1단계 <WEB-INF/web.xml >
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee https://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<!-- The definition of the Root Spring Container shared by all Servlets and Filters -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/root-context.xml</param-value>
</context-param>
<!-- Creates the Spring Container shared by all Servlets and Filters -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
### 서블릿 등록 ###
<!-- Processes application requests -->
<servlet>
<servlet-name>appServlet</servlet-name> = dispatcher
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
= FrontController (WebApplicationContext생성해줌)
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/appServlet/servlet-context.xml</param-value>
= Spring 설정 파일 (다른 특정 경로로 바꿀 수 있음)
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>appServlet</servlet-name>
<url-pattern>/</url-pattern>
" / " 모든 내용을 DispatcherServlet으로 실행
</servlet-mapping>
</web-app>
2단계 <HelloController.java>
package com.bitcamp.mvc;
import java.util.Date;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
### 컨트롤러 설정 ###
@Controller // requestMapping 읽을 수 있도록 @Controller라고 설정
public class HelloController {
// DefaultAnnotationHandlerMapping -> @RequestMapping어노테이션 사용
// 클라이언트 요청 URL과 Controller를 매핑하는 것 (=controller결정)
@RequestMapping("/hello") ** 사용자의 URL 정해주는 것
public ModelAndView hello() {
// FrontController로 전송할 View 경로 & 결과 데이터 -> 저장하고 전달할 객체
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("hello"); ** 이동할 페이지 정해주는 것
// /WEB-INF/views/hello.jsp으로 이동하게함 (ViewResolver에 인해서)
modelAndView.addObject("userName", "Cool"); // request속성으로 공유
modelAndView.addObject("greeting","안녕하세요");
modelAndView.addObject("now",new Date());
return modelAndView;
}
// 추가적으로 설정할 수도 있음
@RequestMapping("/hello1")
public ModelAndView hello1() {
// FrontController로 전송할 View 경로 & 결과 데이터 -> 저장하고 전달할 객체
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("hello"); // /WEB-INF/views/hello.jsp
modelAndView.addObject("userName", "Cool"); // request속성으로 공유
modelAndView.addObject("greeting","안녕하세요");
modelAndView.addObject("now",new Date());
return modelAndView;
}
}
3단계 <servlet-context.xml>
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven />
<!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources directory -->
<resources mapping="/resources/**" location="/resources/" />
### 설정파일에 viewResolver 설정 추가 ###
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
= 응답 view 집합 관리
<beans:property name="prefix" value="/WEB-INF/views/" />
<beans:property name="suffix" value=".jsp" />
= /WEB-INF/view/웅앵웅.jsp -> 모두 뷰로 사용할거임 !!
</beans:bean>
<context:component-scan base-package="com.bitcamp.mvc" />
</beans:beans>
4단계 <index.jsp>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1>index page</h1>
<ul>
<!-- "/hello"라는 controller찾아서 viewPage 겟겟 (requestMapping) -->
<li> <a href="/mvc/hello">/hello</a> </li>
</ul>
</body>
</html>
→ 페이지 이동 <hello.jsp>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1>view: hello.jsp</h1>
<h3>
request으로 받아서 EL로 표현!
userName: ${userName} <br>
greeting: ${greeting} <br>
now: ${now}
</h3>
</body>
</html>
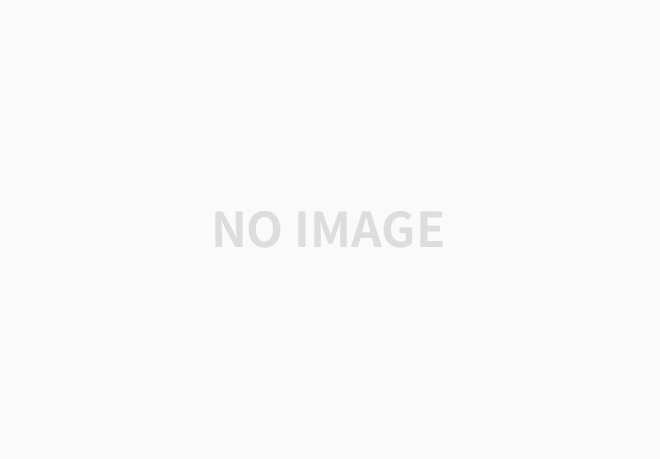
'🔴 Spring' 카테고리의 다른 글
컨트롤러 구현(2) - 커맨드 객체, @ModelAttribute (0) | 2019.11.14 |
---|---|
컨트롤러 구현(1) - 핵심 어노테이션(@Controller, @RequestMapping, @RequestParam, @ModelAttribute) (1) | 2019.11.14 |
AOP - AOP란? AOP vs OOP (1) | 2019.11.13 |
SpringMVC에서의 Annotation, 간단 정리! (0) | 2019.11.13 |
DI(3) - XML없이 자바 코드 기반 설정 (0) | 2019.11.13 |